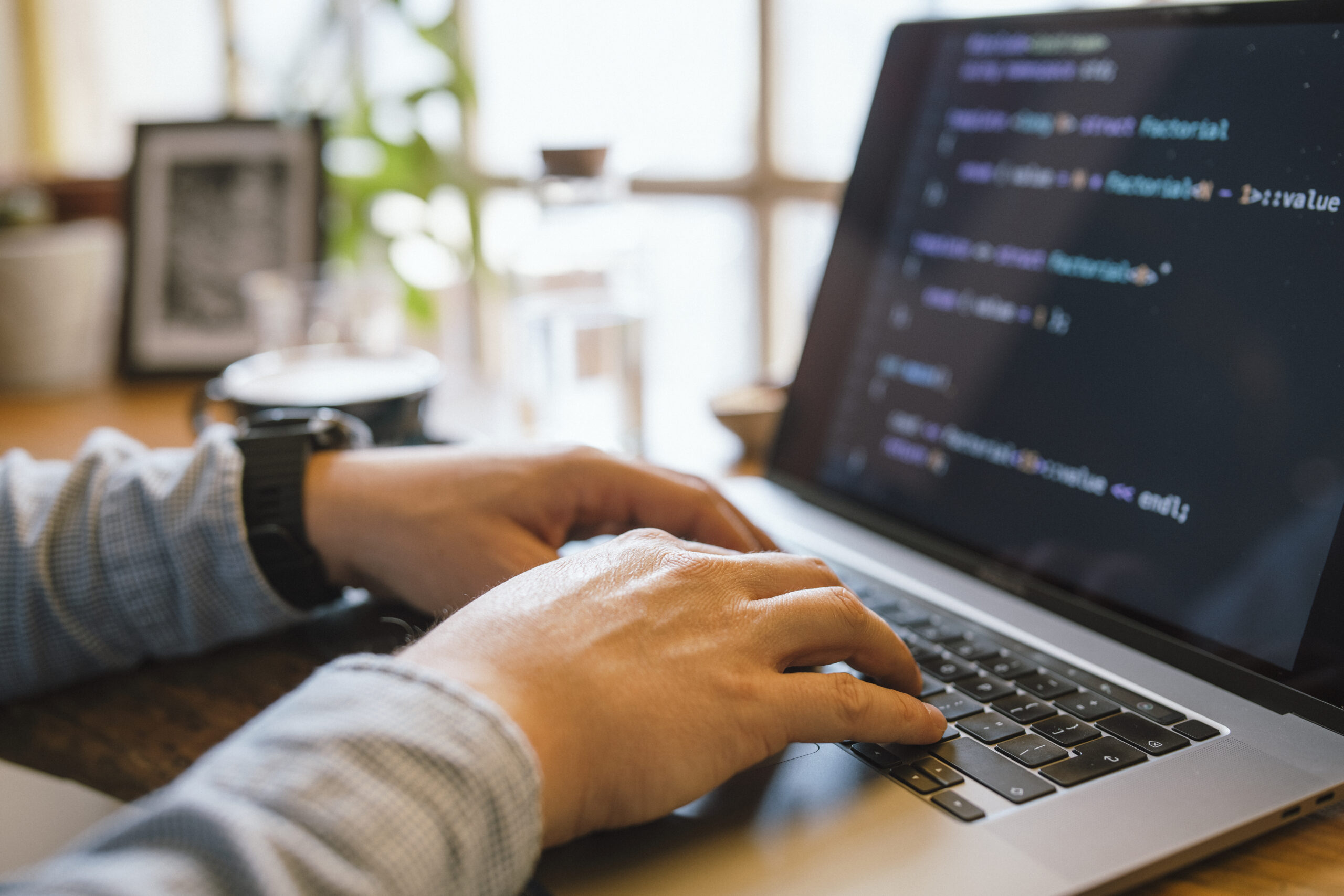
Debugging is Just about the most critical — however typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about knowledge how and why matters go wrong, and Studying to Believe methodically to solve challenges competently. Whether you are a beginner or a seasoned developer, sharpening your debugging skills can save hours of frustration and dramatically improve your productivity. Here are quite a few procedures that will help builders stage up their debugging match by me, Gustavo Woltmann.
Master Your Tools
One of the fastest strategies developers can elevate their debugging abilities is by mastering the tools they use each day. When producing code is a single A part of development, recognizing tips on how to communicate with it successfully during execution is Similarly critical. Modern day improvement environments come equipped with highly effective debugging abilities — but quite a few developers only scratch the surface of what these tools can perform.
Acquire, as an example, an Built-in Growth Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments let you established breakpoints, inspect the value of variables at runtime, move by way of code line by line, and also modify code over the fly. When utilised properly, they Enable you to observe particularly how your code behaves in the course of execution, which is a must have for monitoring down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-finish builders. They permit you to inspect the DOM, watch community requests, check out serious-time efficiency metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into workable duties.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep control in excess of jogging processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Outside of your IDE or debugger, become comfy with Edition Management devices like Git to understand code historical past, uncover the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you recognize your instruments, the more time it is possible to commit fixing the actual difficulty as opposed to fumbling by means of the process.
Reproduce the issue
One of the most critical — and infrequently forgotten — techniques in powerful debugging is reproducing the challenge. Just before jumping into your code or earning guesses, builders want to create a dependable natural environment or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug becomes a activity of probability, usually leading to squandered time and fragile code alterations.
Step one in reproducing an issue is accumulating as much context as possible. Talk to questions like: What steps led to The difficulty? Which surroundings was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater detail you have got, the less complicated it becomes to isolate the precise circumstances underneath which the bug occurs.
When you finally’ve collected plenty of info, seek to recreate the trouble in your neighborhood surroundings. This may indicate inputting the same knowledge, simulating similar consumer interactions, or mimicking procedure states. If the issue seems intermittently, consider composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only aid expose the situation but also avert regressions Down the road.
Occasionally, The problem can be environment-certain — it'd materialize only on particular working devices, browsers, or under certain configurations. Working with applications like virtual machines, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It calls for endurance, observation, in addition to a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. By using a reproducible scenario, You should use your debugging resources much more efficiently, examination prospective fixes securely, and talk far more Plainly using your staff or people. It turns an summary grievance into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most worthy clues a developer has when a thing goes Mistaken. As an alternative to viewing them as irritating interruptions, builders really should study to deal with error messages as immediate communications through the program. They frequently tell you what precisely took place, in which it happened, and sometimes even why it took place — if you understand how to interpret them.
Begin by examining the concept cautiously As well as in total. Numerous builders, particularly when less than time force, glance at the main line and quickly begin earning assumptions. But deeper in the error stack or logs may lie the genuine root trigger. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Break the mistake down into components. Could it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a particular file and line selection? What module or operate triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to know the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some problems are imprecise or generic, and in Individuals scenarios, it’s very important to examine the context during which the mistake happened. Check associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger concerns and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the hood without having to pause execution or move from the code line by line.
A fantastic logging tactic commences with being aware of what to log and at what degree. Frequent logging amounts consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout development, Facts for normal functions (like productive begin-ups), Alert for probable troubles that don’t break the appliance, ERROR for precise challenges, and Deadly when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant information. Too much logging can obscure significant messages and slow down your system. Center on crucial occasions, point out adjustments, enter/output values, and significant choice details within your code.
Structure your log messages Obviously and consistently. Include things like context, including timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t attainable.
Additionally, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with monitoring dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you'll be able to lessen the time it takes to spot troubles, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of one's code.
Consider Similar to a Detective
Debugging is not just a technical activity—it is a sort of investigation. To effectively determine and correct bugs, builders will have to approach the process like a detective fixing a thriller. This frame of mind can help stop working elaborate concerns into manageable areas and observe clues logically to uncover the foundation induce.
Start by gathering evidence. Look at the signs and symptoms of the situation: mistake messages, incorrect output, or effectiveness challenges. Identical to a detective surveys against the law scene, obtain just as much suitable information and facts as you could without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear picture of what’s happening.
Next, variety hypotheses. Talk to on your own: What can be producing this habits? Have any alterations just lately been created for the codebase? Has this problem happened before under identical situation? The purpose is always to narrow down alternatives and establish likely culprits.
Then, examination your theories systematically. Make an effort to recreate the challenge in a very controlled atmosphere. If you suspect a selected operate or component, isolate it and validate if The problem persists. Like a detective conducting interviews, talk to your code inquiries and Allow the effects guide you closer to the reality.
Shell out close notice to modest particulars. Bugs normally conceal in the the very least expected sites—just like a lacking semicolon, an off-by-a single mistake, or even a race situation. Be complete and individual, resisting the urge to patch The difficulty with no fully comprehension it. Temporary fixes may possibly disguise the real challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and turn into more practical at uncovering concealed problems in elaborate methods.
Publish Assessments
Crafting tests is one of the most effective strategies to transform your debugging competencies and General growth effectiveness. Checks don't just help catch bugs early but also serve as a safety net that gives you self-assurance when generating alterations on your codebase. A perfectly-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a dilemma takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated exams can swiftly reveal whether or not a specific piece of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know in which to search, considerably decreasing some time used debugging. Device exams are Particularly useful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-end checks into your workflow. These website support make certain that numerous aspects of your application function alongside one another efficiently. They’re especially practical for catching bugs that come about in sophisticated systems with many elements or products and services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically regarding your code. To check a attribute correctly, you require to comprehend its inputs, envisioned outputs, and edge instances. This volume of knowing The natural way qualified prospects to raised code structure and less bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong first step. When the exam fails constantly, you could concentrate on repairing the bug and check out your check move when The difficulty is resolved. This strategy ensures that the identical bug doesn’t return Sooner or later.
In short, creating assessments turns debugging from the disheartening guessing sport into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive fatigue sets in. You might start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain turns into much less efficient at problem-resolving. A brief stroll, a coffee crack, or maybe switching to a unique process for 10–15 minutes can refresh your aim. Quite a few developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also assist prevent burnout, Primarily through for a longer time debugging sessions. Sitting down in front of a monitor, mentally caught, is not just unproductive but also draining. Stepping absent enables you to return with renewed energy and also a clearer attitude. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re stuck, a very good guideline would be to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver all around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Specifically less than tight deadlines, but it really truly causes more quickly and more practical debugging In the end.
To put it briefly, using breaks is not really a sign of weak spot—it’s a smart method. It presents your brain Place to breathe, increases your perspective, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is a mental puzzle, and relaxation is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just a temporary setback—It really is a possibility to grow like a developer. No matter if it’s a syntax mistake, a logic flaw, or perhaps a deep architectural situation, every one can instruct you some thing worthwhile for those who make an effort to mirror and examine what went wrong.
Commence by asking by yourself a handful of key queries when the bug is solved: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better practices like unit testing, code reviews, or logging? The answers often reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving forward.
Documenting bugs will also be an excellent habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, the way you solved them, and Whatever you realized. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—which you can proactively stay away from.
In team environments, sharing what you've figured out from a bug together with your friends is often Specially effective. Whether or not it’s via a Slack concept, a short generate-up, or A fast understanding-sharing session, helping Some others stay away from the same challenge boosts group performance and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your mentality from stress to curiosity. As opposed to dreading bugs, you’ll start appreciating them as critical areas of your improvement journey. In fact, several of the very best builders aren't those who write best code, but those who continually learn from their faults.
In the end, Every single bug you fix adds a completely new layer in your talent set. So following time you squash a bug, have a second to mirror—you’ll occur away a smarter, far more capable developer on account of it.
Summary
Improving your debugging expertise can take time, practice, and persistence — although the payoff is large. It tends to make you a far more economical, assured, and capable developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be much better at Whatever you do.